Calling unbound Dynamics CRM actions using the Dynamics 365 Web API
We first need to create an unbound action. With unbound I mean “not related to a Dynamics CRM entity”. The image below shows you what a simple unbound action could look like. This action does nothing else but checking the inbound parameter called “Age”. If this “Age” parameter (which will be send to the action using the Web API) is 18 or higher, the action will set the output parameter called “Valid” to “true” and will return this value. If this “Age” parameter is 17 or lower, the action will set the output parameter “Valid” to “false” and will return this value. The image below shows this action.
When we activate this action and browse to (or send a GET request to) “[ORGANIZATION URL]/api/data/v8.1/$metadata”, we will see that metadata about this new action will be exposed by CRM. The image below shows the exposed metadata about the new action:

My action is called “dys_CheckAge”, so for an unbound action, we could now send a POST to “[ORGANIZATION URL]/api/data/v8.1/dys_CheckAge”. We should of course send a JSON object as the body of the POST request, which in our case only has one parameter called “Age”. The object should like this:
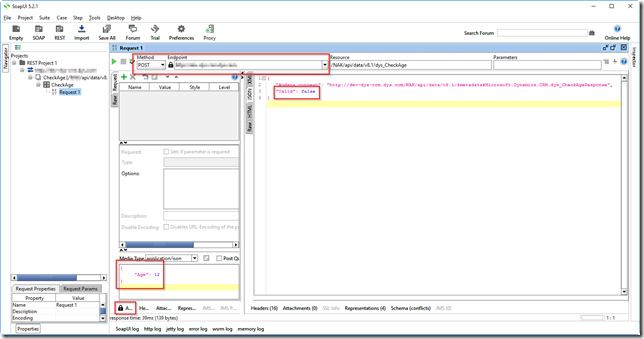
If you send a POST request (using for example SOAPUI) in which the “Age” property has a value of 21, you will see that the action will return the value “true”.
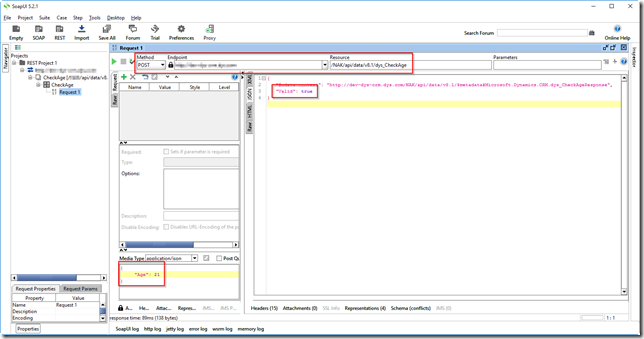
If you take a look at what actually goes over the wire (using for example Fiddler), you will see that it is just a simple POST request to the Web API with a simple body:
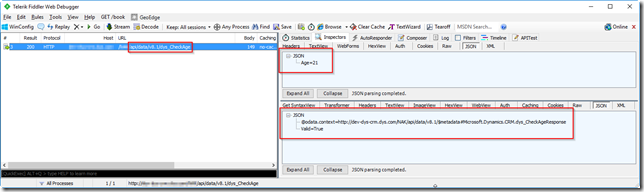
Especially in combination with a Custom Workflow Activity, this can be a very powerful thing to use which can be executed by itself. Calling this action cannot only be done from “inside” CRM using JavaScript, but this can of course also be done from another application (BizTalk / custom .NET application / etc).
My action is called “dys_CheckAge”, so for an unbound action, we could now send a POST to “[ORGANIZATION URL]/api/data/v8.1/dys_CheckAge”. We should of course send a JSON object as the body of the POST request, which in our case only has one parameter called “Age”. The object should like this:
{
"Age": 12
}
If you now send a POST request using for example SOAPUI in which the “Age” property has a value of 12, you will see that the action will return the value “false”."Age": 12
}
If you send a POST request (using for example SOAPUI) in which the “Age” property has a value of 21, you will see that the action will return the value “true”.
If you take a look at what actually goes over the wire (using for example Fiddler), you will see that it is just a simple POST request to the Web API with a simple body:
Especially in combination with a Custom Workflow Activity, this can be a very powerful thing to use which can be executed by itself. Calling this action cannot only be done from “inside” CRM using JavaScript, but this can of course also be done from another application (BizTalk / custom .NET application / etc).
Calling a bound Dynamics CRM actions using the Dynamics 365 Web API
Calling a bound Dynamics CRM action (an action which is related to a Dynamics CRM entity) works almost the same, but works slightly different. The image below shows you what a simple bound Dynamics CRM action could look like:
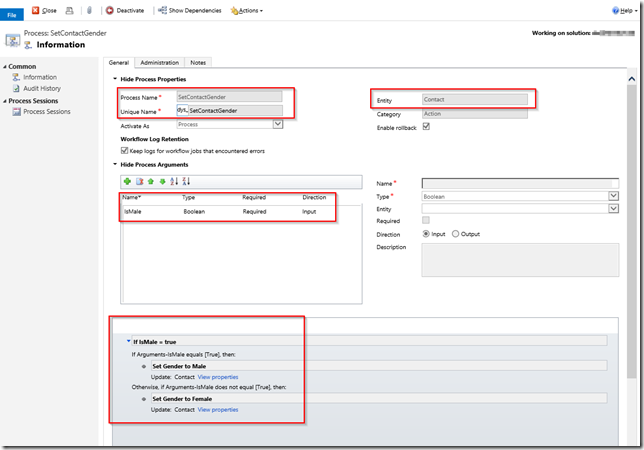
As you can see, the action is bound to the entity “Contact”, has 1 input parameter of type boolean and this input parameter is called “IsMale”. If the input parameter “IsMale” has the value “true”, the “Gender” field will be set to “Male” and the contact will be updated. If the input parameter “IsMale” has the value “false”, the “Gender” field will be set to “Female” and the contact will be updated.
As soon as you activate this action and browse to (or send a GET request to) “[ORGANIZATION URL]/api/data/v8.1/$metadata”, we will see that metadata about this new bound action will also be exposed by CRM. The image below shows the exposed metadata about the new action:

The difference between calling an unbound action and a bound action is the URL. As said above, an unbound action can be access by “[ORGANIZATION URL]/api/data/v8.1/[ACTION-NAME]” and a bound action can be accessed / called by using the following URL: “[ORGANIZATION URL]/api/data/v8.1/ENTITYNAME(ENTITY-ID)/Microsoft.Dynamics.CRM.[ACTION-NAME]”.
If you would now send a POST request (using for example SOAPUI) in which the “IsMale” property is set to “true”, you will see that the action will set the contact “Gender” to “Male”.
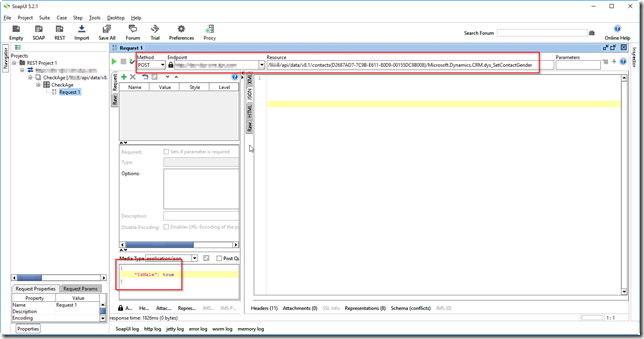
If you again would send a POST request (using for example SOAPUI) in which the “IsMale” property is set to “false”, you will see that the action will set the contact “Gender” to “Female”.
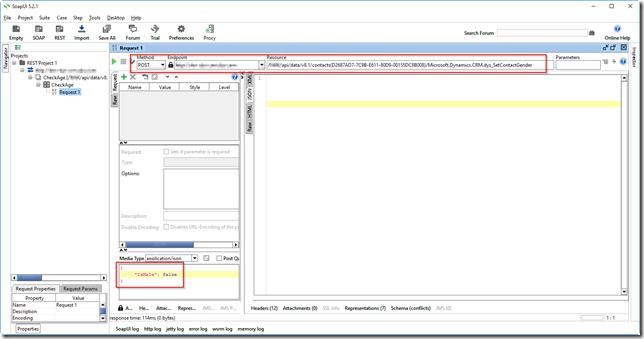
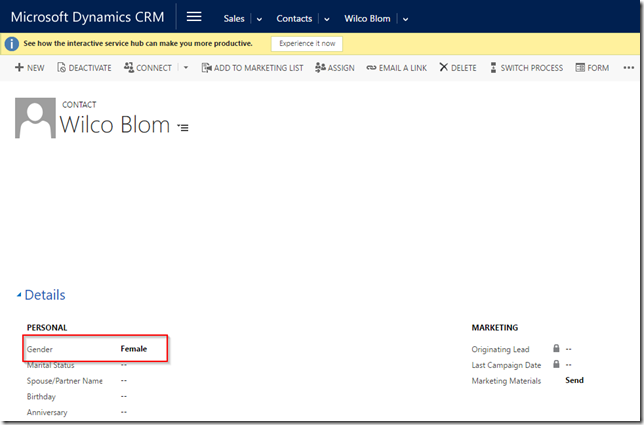
As you can see, the action is bound to the entity “Contact”, has 1 input parameter of type boolean and this input parameter is called “IsMale”. If the input parameter “IsMale” has the value “true”, the “Gender” field will be set to “Male” and the contact will be updated. If the input parameter “IsMale” has the value “false”, the “Gender” field will be set to “Female” and the contact will be updated.
As soon as you activate this action and browse to (or send a GET request to) “[ORGANIZATION URL]/api/data/v8.1/$metadata”, we will see that metadata about this new bound action will also be exposed by CRM. The image below shows the exposed metadata about the new action:
The difference between calling an unbound action and a bound action is the URL. As said above, an unbound action can be access by “[ORGANIZATION URL]/api/data/v8.1/[ACTION-NAME]” and a bound action can be accessed / called by using the following URL: “[ORGANIZATION URL]/api/data/v8.1/ENTITYNAME(ENTITY-ID)/Microsoft.Dynamics.CRM.[ACTION-NAME]”.
If you would now send a POST request (using for example SOAPUI) in which the “IsMale” property is set to “true”, you will see that the action will set the contact “Gender” to “Male”.
If you again would send a POST request (using for example SOAPUI) in which the “IsMale” property is set to “false”, you will see that the action will set the contact “Gender” to “Female”.
Calling a Dynamics CRM actions using JavaScript and the Dynamics 2016 Web API
A Dynamics CRM action can also contain business logic which can be triggered (called) using JavaScript and which helps the user (client-side) during data entry. One of the actions we created above was the “CheckAge” action which expects an “Age” property and returns the value “true” if the age is equal or above 18 (the JSON object returned will have a “Valid” property of type boolean). In all other cases it will return the value “false”. This action can be called using the following JavaScript:
"use strict";
var Dys = window.Dys || {};
Dys.ValidateField = function (context) {
var data = {
"Age": context.getEventSource().getValue()
};
//dys_CheckAge is the name of the custom unbound action in this case
Dys.CallAction("dys_CheckAge", data, Dys.ValidateFieldUsingActionCallBack);
}
Dys.CallAction = function (query, data, callback) {
var url = Xrm.Page.context.getClientUrl() + "/api/data/v8.0/" + query;
var req = new XMLHttpRequest();
req.open("POST", url, true);
req.setRequestHeader("Accept", "application/json");
req.setRequestHeader("Content-Type", "application/json; charset=utf-8");
req.setRequestHeader("OData-MaxVersion", "4.0");
req.setRequestHeader("OData-Version", "4.0");
req.onreadystatechange = function () {
if (this.readyState == 4) {
req.onreadystatechange = null;
if (this.status == 200) {
callback(req.response);
} else {
var error = JSON.parse(this.response).error;
alert(error.message);
}
}
};
req.send(window.JSON.stringify(data));
}
Dys.ValidateFieldUsingActionCallBack = function (response) {
alert("The value is: " + JSON.parse(response).Valid);
}
Summary
Although this example shows very simple scenario’s, the possibilities are clear:- You are able to execute pieces of reusable (business) logic from inside or outside CRM as if it were a “custom service”.
- Althoug creating and updating CRM data is possible without using the Dynamics CRM actions as well (by simply sending a POST the the Web API), using the Dynamics CRM actions allow you to apply business logic before anything is created / updated.
- Complex business logic can be executed if you create a Custom Workflow Activity (CWA) which is used inside your Dynamics CRM action.
- A Dynamics CRM action also enables you to decouple the inside of CRM from the outside of CRM. With this I mean that you can keep your input parameters the way ther are, but do the translation (to a changed fieldname for example) in the action. This way, the outside world can reuse your action the way they are used to, while you shuffle things around within Dynamics CRM.